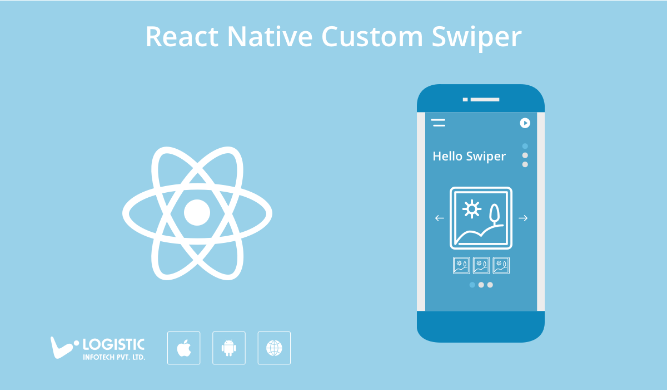
In this article, you will learn about custom swiper integration with your React native application using flatlist. Mostly when you are dealing with photo app you may need a feature which will allow you to swipe easily on screen with different images.
From the inception of your app idea, you plan to support both iOS and Android devices, but it does not make sense to spend time learning, implementing and optimizing separate swiper code for each of the platforms
During the development of one of my application, I need this kind of component. With React Native I have two option for this either create bridging and use native support for swiper or implement your own custom swiper. Finally, I have chosen a way to create own swiper using flatlist so it will help other developers who are looking for this kind of functionality for their app development.
Here you will find pure Javascript based react-native-custom-swiper library that uses native drivers for fluid transitions. React Native Swiper adopts this approach that avoids any required knowledge in Objective C / Swift / Java / Kotlin, allowing you to focus more on the app experience.
Implementing custom swiper with your new React Native Application
Here I am presuming that you are aware of React Native development and having preconfigured react-native set up on your system. Let’s create a new application using below command.
react-native init <Your Application Name> cd <Your Application Name> npm install
Once your app is created, you need to run below command to add custom swiper library with you project.
npm i react-native-custom-swiper
Once library is added, let’s open your App.js file and set up custom swiper as shown in below code.
* Import import React, { Component } from "react"; import Swiper from "react-native-custom-swiper";
* Initialization of your slide data constructor(props) { super(props); this.state = { imgArray: [ require("./src/Resource/Images/bridge.jpg"), require("./src/Resource/Images/Hill.jpg"), require("./src/Resource/Images/animal.jpg"), ( You can use your own data here ) ], currentIndex: 0, }; }
You may need to put images in your resource folder and provide proper path in image array.
Implement custom swiper for view
Let’s add Swiper as shown in below code.
render() { return ( <View style={styles.container}> <Swiper style={{ flex: 1 }} currentSelectIndex={0} swipeData={this.state.imgArray} renderSwipeItem={this.renderImageSwipeItem} onScreenChange={this.screenChange} /> </View> ); }
You are also able to customise your SwipeItem like below.
renderImageSwipeItem = item => { return ( <View> <Image style={{ height: 340, width: width }} source={item} resizeMode="contain" /> </View> ); };
Handle swiper position change.
screenChange = index => { console.log("index when change :=> \n", index); this.setState({ currentIndex: index }); };
Finally render method of your App.js file will look like below by combining all above steps.
render() { return ( <SafeAreaView style={styles.container}> <View style={{ width: width, height: height }}> <View style={{ backgroundColor: "rgb(221,244,253)", width: width }}> <Text style={{ marginTop: 60, fontWeight: "bold", textAlign: "center", fontSize: 26, color: "black" }} > RN Custom Swiper </Text> <Text style={{ marginTop: 5, textAlign: "center", fontSize: 23, fontStyle: "italic", color: "black" }} > Current Index : {this.state.currentIndex} </Text> </View> <Swiper currentSelectIndex={0} backgroundColor="rgb(221,244,253)" swipeData={this.state.imgArray} renderSwipeItem={this.renderImageSwipeItem} onScreenChange={this.screenChange} /> </SafeAreaView> ); }