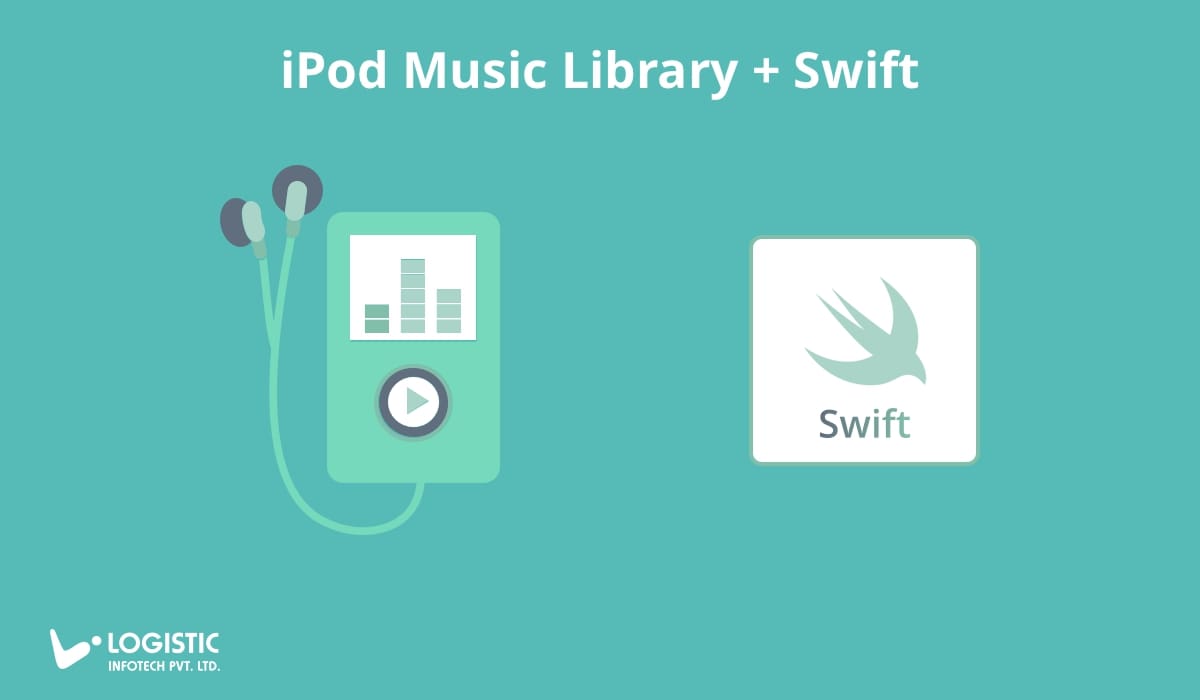
Generally, Apple is very strict regarding access to local storage on your iPhone. Specifically, you can’t access the audio file stored in your iPod library to directly in your application as a raw format. In this article, you will learn how to import an audio file from your iPod Music Library.
We will accomplish this using MPMediaPickerController. Which will allow you to choose audio files from iPod Library and provide a list of items you have selected as MPMediaItemCollection.
Play iPod Music Library
If you are not looking for any other operation and just want to play on your end then it will be accomplished by below code and it will play all items you have selected in a queue.
let player = MPMusicPlayerController.applicationMusicPlayer player.stop() player.setQueue(with:mediaItemCollection) delay(0.2) { player.play() }
But if you are looking for any other purpose and want to save them in your document directory, then you need to follow few more steps to export raw file from MPMediaItemCollection as written in below code snippets.
This will be accomplished with the help of AVAssetExportSession. You need to iterate same thing for all items you have selected from iPod Library. To export file you first need to find AssetURL. You can get that AssetURL using these two lines of code: –
let item: MPMediaItem = mediaItemCollection.items[0] let pathURL: URL? = item.value(forProperty: MPMediaItemPropertyAssetURL) as? URL
Therefore to initialize export session you need to set assets, export preset name and output file type. You can set them accordingly. Here in this article, I have used AVAssetExportPresetPassthrough for export preset name and output file type as per its own type.
Export audio file to Document directory
In short, to store exported file you need to specify storage path as below.
let documentURL = try! FileManager.default.url(for: .documentDirectory, in: .userDomainMask, appropriateFor: nil, create: true) let outputURL = documentURL.appendingPathComponent("custom.m4a") //Delete Existing file do { try FileManager.default.removeItem(at: outputURL) } catch let error as NSError { print(error.debugDescription) } exportSession?.outputURL = outputURL
Finally, here you can see code snippets to export media file from iPod Music Library.
exportSession?.exportAsynchronously(completionHandler: { () -> Void in if exportSession!.status == AVAssetExportSession.Status.completed { print("Export Successful") } else { print("Export failed") print(exportSession!.error) } })
Finally, you have raw file stored in your document directly and you can use that file for any operation as per your requirements. Here you can find code with simple music player implementation and it will play all exported files from your local directory.