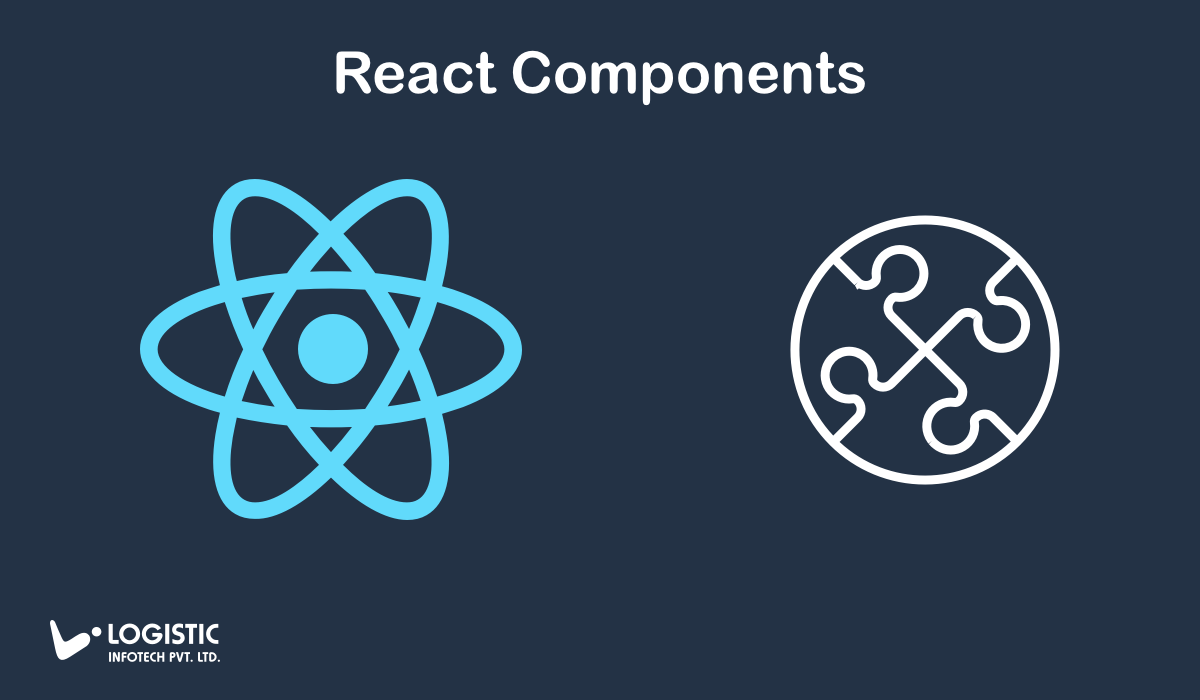
Recently React is the most popular javascript framework for building a front-end. React is a rich library with thousands of components. In this article, you will learn about React Component, it’s types and how to use them. Different type of component has a different responsibility. There are many ways to create React Component e.g. using state, without a state(stateless), Pure Component, etc…
Usually, there is confusion with types of component and their usage. Most of the developers are confused with the difference between stateless and stateful components and which should be used for better performance. Here in this article, you will learn how exactly stateful and stateless component will work and which should you use.
Stateless Component
A React stateless components simply returns a value which you input in your view. There are no states but methods may be there. It is very easy to create maintainable and readable code. The stateless component will break the components into small parts and pass data through props. This is also called as Pure Function. There is no ‘this’ keyword there. And it also has no lifecycle method like componentDidMount.
How it works?
The stateless component takes props as an argument and returns a new react component. When rendering stateless component you need to just call your stateless component function and pass props.
Let’s understand with example
// Stateless component const MyStateLessComponent = ({ title, subtitle }) => { return ( <View> <Text>{title}</Text> <Text>{subtitle}</Text> </View> ); }; export default MyStateLessComponent;
// Stateless component using Arrow function const MyComponent = ({Message}) => ( <Text>{Message}</Text> )
// Implementing Stateless component in your class <MyStateLessComponent title ={this.state.title} subtitle ={this.state.subtitle} />
This is how stateless function works. As you can see there is no local state in the component. You need to pass text through props and it will display the text which you sends. Stateless components are good for small UI view.
Stateful Components
Generally, a React stateful component has the class syntax and it extends the React components base class. With stateful components, you can access the life cycles methods e.g. render, constructor, setState, componentDidMount, etc… It is also called an Impure Function.
How it works?
Stateful components basically depend on the state which you defined inside the constructor. When you need to change the value you need to change the state’s value using this.setState({}). And if states value changed then your component should automatically update.
Let’s understand with example
import React from "react"; import { StyleSheet, Text, View, ActivityIndicator } from "react-native"; class App extends React.Components{ constructor(){ super(); this.state = { isLoading : false, value:0, } } increaseValue = () => { this.setState( value : this.state.value + 1 ); } render(){ return( <View style={styles.container}> <Text style={styles.text}> Stateful Component!! </Text> <TouchableOpacity onPress={this.increaseValue}> <Text style={styles.text}>Increase</Text> </TouchableOpacity> </View> ) } } export default App;
Above example is stateful because you first initialize state then you have an increaseValue() method in which the value of your state is changes and handle the text value.
Pure components
This is generally used for optimizing the application. The pure component also used for increasing performance because it will reduce the number of operations in the application. Pure components working is same as shouldComponentUpdate. In shouldComponentUpdate, we check manually to re-render or not but in pure component check internally and increase the performance of our application. It is the simplest and fastest component we can write. Pure component avoids all previous rendering request.
class example extends PureComponent{ render(){ return( <Text style={styles.text}>{this.props.text}</Text> ) } }
Let’s differentiate both stateless and stateful component
So the confusion is which should you use? Basically, there is an insignificant difference in render time. But if you have more classes then you need to use Stateless component. There is a main difference is the amount of code. If you need to use lifecycle methods then go with the stateful component rather than stateless, but if lifecycle methods are not required then stateless is a better one.
I hope you enjoyed this article. Thanks for reading