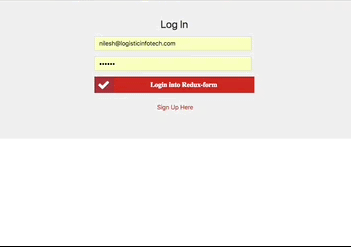
While dealing with an ordinary way of react form validation I have to keep the value of each form component in the state. It’s tedious to manage each and every input elements of form. Thanks to Redux Form which provides the best way to deal with this headache.
React Redux Form provide very easy and best way to implement validation with any complex form development and save lots of time.
Finally, I have found a solution to overcome the above issue using Redux Form. It provides the best way to deal with complex form implementation as it stores form state directly in store.
Let’s assume that you are familiar with Redux which uses the concept of unidirectional data flow.
What is Redux Form?
We can say Redux Form is a group of controlled components which makes implementation of very complex form or custom form very much easy. Its store all of its state in Redux store.
Installation
npm install --save redux-form --save //Import it in your component import { Field, reduxForm } from "redux-form";
Redux Form basic terms.
Here I am going to explain basic terms and how to add in your code.
1 ) formReducer functions is required for how to update redux store according to changes done in form which are provided by Redux actions.
import { combineReducers } from "redux"; import { reducer as formReducer } from "redux-form"; export default combineReducers({ form: formReducer, });
2) reduxForm() function collect configuration objects and return new functions which wrap your form component and bind with redux dispatch actions.
Form Component communicate with redux store, its wrapped by reduxForm() which provide props to form and handle form submit event.
3) <Field/> components are used for input components to redux-form logic
<Field id="login-email" name="lemail" type="email" className="form-control" component={renderField} label="Email" onChange={this.login} />
Here I have implemented Redux Form which requires me to set up action creators, reducers in sort we can say most of redux will be used.
Login Form Using Redux-Form
Let’s take a look at the code and see how I have developed simple login and registration form using Redux Form.
<form onSubmit={this.submit} method="POST" className="m-t mx-auto"> <h2 className="mb-3 text-center font-weight-normal">Log In</h2> <div className="mb-3"> <div className="row d-flex justify-content-center"> <div className="col-sm-12"> <Field id="login-email" name="lemail" type="email" className="form-control" component={renderField} label="Email" onChange={this.login} /> </div> </div> </div> <div className="mb-3"> <Field id="login-password" name="lpassword" type="password" className="form-control" component={renderField} label="Password" onChange={this.login} /> </div> <div className="form-group float-left"> <a href="javascript:void(0)" className="forgot-pass"> {/* Forgot Password? */} </a> </div> <div className="hb-social-icons-wrapper mobile-mx-1"> <button type="submit" disabled={submitting | invalid} id="login-btn" className="btn p-0" > <div className="login-boycott-bg-btn social-bg bg-primary d-flex justify-content-between align-items-center hb-fix-btn m-0 hb-login-fix-hieght"> <div className="float-left social-icon-bg login-boycott-bg hb-login-fix-hieght"> <i className="fa fa-check" aria-hidden="true" /> </div> <div className="hb-btn-text login-boycott mx-auto" id="btnLogin"> Login into Redux-form <i id="loading-submit" className="fa fa-circle-o-notch fa-spin ml-3 p-0 d-none" /> </div> </div> </button> <div className="align-items-start pt-1 " id="verification-error" /> </div> </form>
Here is code snippiest for validation I have used.
const validate = values => { const errors = {}; if (!values.lpassword) { errors.lpassword = "Password is required."; } else if (values.lpassword.length > 8) { errors.lpassword = "Must be 8 characters or less"; } if (!values.lemail) { errors.lemail = "Email is required."; } else if (!/^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$/i.test(values.lemail)) { errors.lemail = "Invalid email address"; } return errors; };
Registration Form Using Redux Form
Let’s take a look at the code and see how I have developed simple registration form using Redux Form. In this form, I have added date input for birthdate and radio button for gender selection. Because they are special cases and mostly need special care. I have found this solution after working more than 4-5 hours on this.
<form id="register-form" onSubmit={this.submitRegister} method="POST" name="register-form"> <h2 className="mb-0 font-weight-normal text-center">Sign Up</h2> <div className="row no-gutters hb-register"> <label htmlFor="name" className="col-md-12 col-form-label text-md-left"> Full Name </label> <div className="col-md-6 col-sm-6 mobile-pr-0 pb-2 hb-padding-right-8"> <Field id="fname" type="text" className="form-control" placeholder="First Name" name="fname" onChange={this.register} component={renderField} /> </div> <div className="col-md-6 col-sm-6"> <Field id="lastname" type="text" className="form-control" placeholder="Last Name" name="lastname" onChange={this.register} component={renderField} /> </div> </div> <div className="row hb-register"> <label htmlFor="email" className="col-md-12 col-form-label text-md-left" style={{ marginLeft: "-13px" }} > Your Email Adress </label> <Field id="reg-email" type="email" className="form-control" name="email" width="453px" onChange={this.register} component={renderField} /> </div> <div className="row hb-register password_criteria"> <label htmlFor="password" className="col-md-12 col-form-label text-md-left" style={{ marginLeft: "-13px" }} > Create Your Password </label> <Field id="reg-password" type="password" className="form-control" name="password" width="453px" onChange={this.register} component={renderField} /> </div> <div className="row hb-register"> <label htmlFor="password" className="col-md-12 col-form-label text-md-left" style={{ marginLeft: "-13px" }} > Confirm Password </label> <Field id="reg-confirm-password" type="password" className="form-control" name="rconfirmpassword" width="453px" onChange={this.register} component={renderField} /> </div> <div className="row hb-register"> <label htmlFor="password" className="col-md-12 col-form-label text-md-left " style={{ marginLeft: "-13px" }} > DOB </label> <Field id="dob" className="form-control" name="dob" width="453px" selected={this.state.date} onChange={this.dateChanged} component={renderDatePicker} /> </div> <div className="row hb-register gender" style={{ marginTop: "7px" }}> <label style={{ width: "66px" }}> Gender:</label> <RadioGroup name="gender" value="3"> <Radio value="male" style={{ width: "150px" }} radioClass="iradio_square-blue" increaseArea="20%" label="<span class='label1' style='width:15px;'>Male</span>" /> <Radio value="female" style={{ width: "150px" }} radioClass="iradio_square-blue" increaseArea="20%" label="<span class='label1'>Female</span>" /> </RadioGroup> </div> <div className="text-center mb-3 text-lowercase"> <small /> </div> <div className="mb-2 mobile-mx-1"> <div className="hb-social-icons-wrapper text-center"> <button type="submit" id="btn-submit" className="btn p-0" disabled={submitting | invalid} > <div className="login-boycott-bg-btn social-bg bg-primary d-flex justify-content-between align-items-center hb-fix-btn m-0 hb-login-fix-height"> <div className="float-left social-icon-bg login-boycott-bg hb-login-fix-height"> <i className="fa fa-check" aria-hidden="true" /> </div> <div className="hb-btn-text login-boycott mx-auto" id="btnSignup"> Sign Up For <i id="loading-submit-reg" className="fa fa-circle-o-notch fa-spin ml-3 p-0 d-none" /> </div> </div> </button> </div> </div> <div className="d-flex justify-content-center"> <label> Already Have An Account?</label> <Link to="/">Log in Here</Link> </div> </form>
Here I have written date change method separately for birthdate change in form.
dateChanged = d => { this.setState({ ["date"]: d }); let loginCopy = { ...this.state }; loginCopy["date"] = d; this.setState(loginCopy); // this.setState({ ["dob"]: d }); console.log("state: ", this.state); };
Let me write a small summary here, in simple words I can say that using Redux Form I have control of all form data and its behaviors.
I have created a Github repository with all implementation we discussed above. You will enjoy working with Redux-Form implementation. Here you can find code with implementation.