It is essential for each and every application to sync with the backend server and this communication will be done by calling APIs from the application side. There are many ways to call API from the application side to server. Here I have tried to explain how to fetch data in React Native application from the backend server and which one will be best to use.
Here are options being used for communication.
1. Fetch API
2. HTTP Request
Fetch API
It fetches data asynchronously across the network and provide promise-based and returns a response object. Fetch use same concept of Request and Response which involved with network requests.
How to call Fetch API?
Here is sample code for your reference.
fetch(“https://youtserverurl.com/api/?records=10”) .then(res => res.json()) .then(res => { console.log(“Server response :- \n”, res); }) .catch(error => { console.log(“Error from server :- \n”, error); });
Fetch API will work for text as well as with form-data request and return blob, text or JSON as a response.
Fetch is very easy to implement and use but fetch didn’t feel so attractive for the larger project where we need to cancel request, upload progress or request timeout. In addition to this, it does not provide better https status handling or error handling.
Here is screenshot for simple fetch request call with response without applying json().
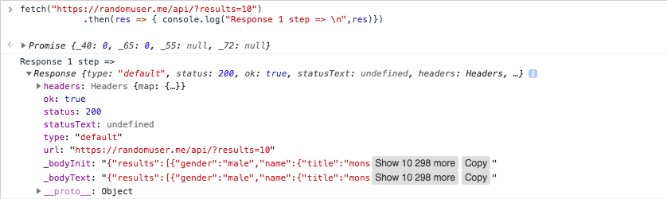
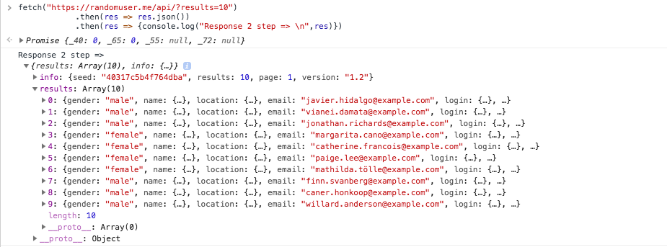
HTTP Requests using Axios
Here I have considered Axios library for comparing with fetch request. There are many libraries available in NPM for HTTP request but Axios is one of the best which I have chosen here.
Axios is a Javascript library used to make HTTP requests and it supports the Promise API that is native to JS ES6 which provide automatic transform of data in JSON format. Axios supports canceling request, timeout and it will also provide uploading progress for any file uploading.
How to fetch data using Axios?
The first step in using Axios is installing Axios and import in your JS file where you want to implement this library for data fetching.
npm install axios --save import axios from "axios"
Here is sample code for API call using Axios.
axios .get(“https://randomuser.me/api/?results=10”) .then(function(response) { Constants.debugLog(“Response Data :=> \n” + JSON.stringify(response)); }) .catch(function(error) { Constants.debugLog(“Response Error :=> \n” + JSON.stringify(error)); });
Above API will return a list of Users randomly, you can easily check in the console as shown in below screenshot. Axios will provide JSON response so we don’t need to convert it to JSON object.
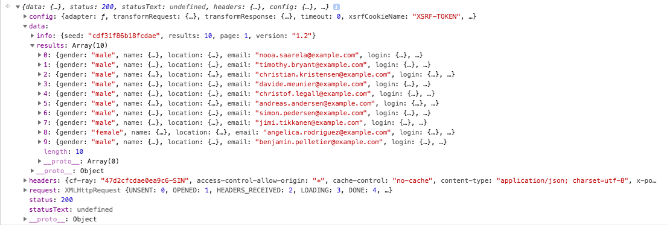
As we discussed above here is a list of features,
1. Progress Report: Axios provide progress while calling API, especially for file uploading as you can see in below code block.
axios.post(‘url’, data, { onUploadProgress: ({ total, loaded }) => { // update progress }), })
2. Error handling : Axios provide the best way to handle errors by response code while fetch will not provide this option. This is an inconvenience in most cases since all your previous assumptions of what a response would look like are no longer valid.
Most often, when receiving an erroneous response from the server you’d want to abort the processing pipeline as soon as possible, and switch to an error handling case.
Here is an example how to handle errors using response code.
3. Cancel requests and timeout feature : Axios provide the best way to handle to cancel request and options to set a timeout for calling API.
fetch(url) .then(reponse => { if (!(status >= 200 && status < 300)) { return Promise.reject(new Error(“Invalid response status”)); } return response; }) .then(response => response.json()) .then(/* normal processing */) .catch(/* error handling */);
Finally, the .fetch() method is a great step in the right direction of getting HTTP requests native in ES6, but just know that if you use it there are a couple of gotchas that might be better handled by third-party libraries like Axios.
You can find other interesting blogs for Mobile Application Development here.